As nice as event-driven programming can be within the context of WordPress’ hook system, one of the challenges is preventing code from executing every single time the hook is called.
Multiply this across however many callbacks spread across however many files and general functionality registered with the hook and you may end up affecting performance and/or executing code that has no need to be run.
If your plugin’s bootstrap registers a callback with a WordPress hook, considering using static variables to prevent code from being called unnecessarily more than once.
Static Variables in Plugin Bootstrap Files
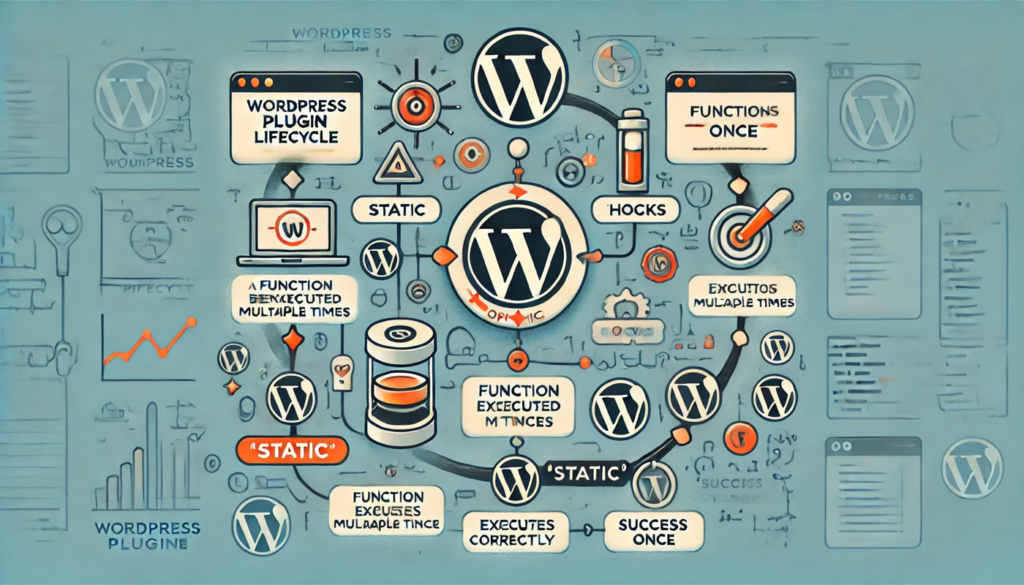
For example, say you’re writing a function that fires during the init
action but something happens in WordPress core that triggers the init
action to fire again consequently causing your code to fire once again even though it’s unneeded.
So having an static $initialized
flag works like this:
But if not, static
variables can be useful in this scenario because static
variables maintain state between calls whereas regular variables are reinitialized every time the function fires. This means a static
variable retains its value across multiple calls to the function.
One way this can happen is in a plugin’s bootstrap. Case in point: Say your plugin is registered with the init
action and then it sets up a registry which in turn instantiates a set of subscribers that register services. Repeating this every single time init
is fired is unnecessary.
Static Variables and Plugins
add_action( 'init', 'tm_acme_function', 100);
function tm_acme_function() {
static $initialized = false;
if ( $initialized ) {
return;
}
$initialized = true;
// ... set up the rest of the function.
}
Here’s a way to manage this:
- prevents redundant execution,
- optimizes performance by avoiding running unnecessary code (especially as it relates to registering duplicate functionality, running multiple queries, or trashing data unintentionally).
And because of that, this: